How to send text messages using C#
by TextPort
Posted: December 17, 2019
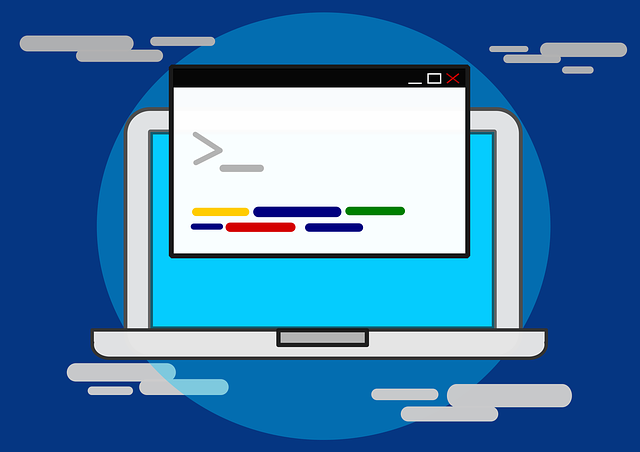
TextPort’s REST SMS API is an easy way to send text messages using C#. This article guides you through setting up an account, writing the code, and sending texts using C# in as little as 15 minutes.
Set up an account
The first step is to set up an account. TextPort offers a 15-day free trial. No credit card or money is needed to get started. Visit the free trial page to set up an account and get a free virtual mobile number. The number you choose will be the number that your texts will be sent and received from.
Add an API Application
Once your account is set up, go to the API Applications page. Click your username in the top-right corner, then select API Applications.
Assign a name to your your application. TextPort will generate an API token and API key. These are your API username and password. They will be passed in the HTTP header using basic authentication. See the code samples below to see how these are applied.
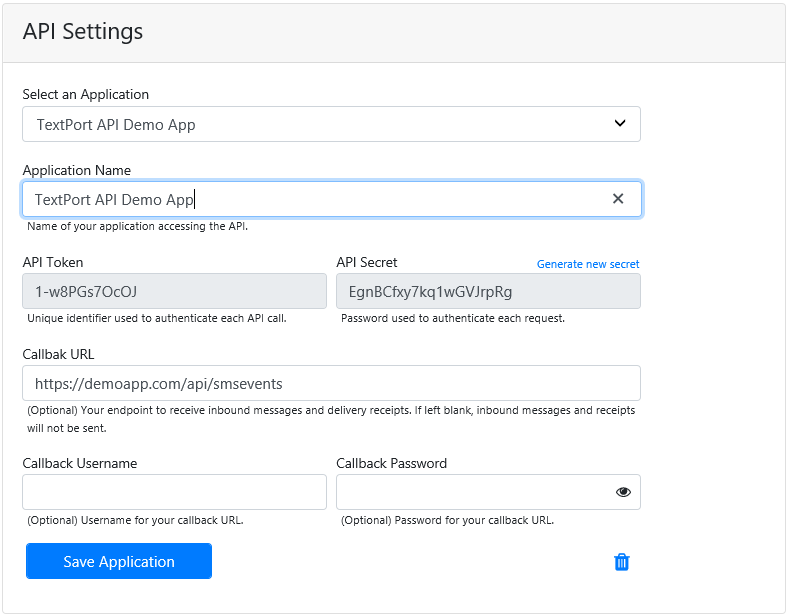
Associate your API Application with your Virtual Number
The next step is to assign the API application that you just created to your virtual number. Navigate to your Numbers page. Here you will see your virtual number. Click the Apply link to display the Assign API Application dialog.

Select the API application that you created in the step above and click Apply.
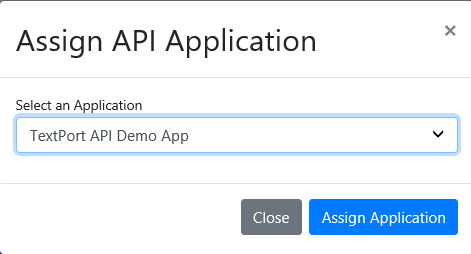
Your Numbers page should now look like this.

Now to start writing some code.
Here we'll demonstrate how to send a text message using C# with both the .NET WebClient and the newer HttpClient. There's no preferred method to use, so it's simply a matter of personal preference. An alternative would be to use the popular RestSharp client for .NET. This post outlines how to send SMS messages using RestSharp.
Using the WebClient class
using System.Net;
public void SendSMSUsingWebClient()
{
string jsonData = @@"[ { 'From': '14045551212', 'To': '12065551650', 'MessageText': 'Hello world.' } ]";
string apiToken = "63871-opY49WGQ8s";
string apiSecret = "GkRMr2ffrgtvns9g5mHe";
using (WebClient client = new WebClient())
{
// Add the apiToken and apiSecret as credentials
client.Credentials = new NetworkCredential(apiToken, apiSecret);
client.Headers.Add(HttpRequestHeader.ContentType, "application/json");
// Send the request
string response = client.UploadString(new Uri("https://api.textport.com/v1/messages/send"), "POST", jsonData);
}
}
Using the HttpClient class
using System.Text;
using System.Net.Http;
public async Task SendSMSUsingHttpClient()
{
string jsonData = @@"[ { 'From': '14045551212', 'To': '12065551650', 'MessageText': 'Hello world' } ]";
// Initialize an instance of the HTTP client
using (HttpClient client = new HttpClient())
{
client.BaseAddress = new Uri("https://api.textport.com");
HttpRequestMessage request = new HttpRequestMessage(HttpMethod.Post, "/v1/messages/send");
// Add the credentials using basic authentication. This will generate an HTTP header like:
string apiToken = "63871-opY49WGQ8s";
string apiSecret = "GkRMr2ffrgtvns9g5mHe";
byte[] byteArray = new UTF8Encoding().GetBytes($"{apiToken}:{apiSecret}");
client.DefaultRequestHeaders.Authorization = new System.Net.Http.Headers.AuthenticationHeaderValue("Basic", Convert.ToBase64String(byteArray));
// Add the JSON content to the request
request.Content = new StringContent(jsonData, Encoding.UTF8, "application/json");
HttpResponseMessage response = await client.SendAsync(request);
string jsonResult = await response.Content.ReadAsStringAsync();
}
}
Regardless of which of the above methods you use, the end result will be the same. If the request is successful you'll receive an HTTP 200 (OK) response back, with a JSON (or XML) payload confirming the details of the messsage and the remaining balance.
[
{
"From":"14045551212",
"To":"12065551650",
"MessageText":"Hello world",
"Status":"OK",
"ErrorMessage":"",
"MessageId":10488785,
"Balance":48.2500
}
]
What if I'd prefer to use XML instead of JSON?
If you prefer to use XML over JSON, simply change the value of the Content-type header key to "text/xml". TextPort will accept and return both XML and JSON payloads.
Conclusion
That's all there is to it. It's that simple to send SMS messages using C#. Click here for more information on TextPort's SMS API documentation and code samples.
Recent Articles
- 7 Benefits of SMS Marketing
- Send Texts Online in 5 Easy Steps
- 5 Ways to Send Text Messages Online
- 5 Rules for Effective SMS Marketing in 2020 - and Beyond
- Sending Text Messages from Foreign Countries to the United States
- Send Picture Messages from Your Computer
- How to send text messages using RestSharp
- How to send text messages using C#
- How to Send and Receive Text Messages Online
- Effective SMS Marketing